Public Member Functions |
Static Public Member Functions |
Protected Attributes |
List of all members
App\Models\Location Class Reference
Inheritance diagram for App\Models\Location:
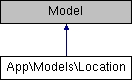
Public Member Functions | |
users () | |
assets () | |
assignedassets () | |
parent () | |
childLocations () | |
scopeTextsearch ($query, $search) | |
Query builder scope to search on text. More... | |
scopeOrderParent ($query, $order) | |
Query builder scope to order on parent. More... | |
Static Public Member Functions | |
static | getLocationHierarchy ($locations, $parent_id=null) |
static | flattenLocationsArray ($location_options_array=null) |
Protected Attributes | |
$dates = ['deleted_at'] | |
$table = 'locations' | |
$rules | |
$injectUniqueIdentifier = true | |
$fillable = ['name'] | |
Detailed Description
Definition at line 10 of file Location.php.
Member Function Documentation
App\Models\Location::assets | ( | ) |
Definition at line 48 of file Location.php.
App\Models\Location::assignedassets | ( | ) |
Definition at line 53 of file Location.php.
App\Models\Location::childLocations | ( | ) |
Definition at line 63 of file Location.php.
|
static |
Definition at line 96 of file Location.php.
static flattenLocationsArray($location_options_array=null)
Definition: Location.php:96
|
static |
Definition at line 68 of file Location.php.
static getLocationHierarchy($locations, $parent_id=null)
Definition: Location.php:68
App\Models\Location::parent | ( | ) |
Definition at line 58 of file Location.php.
App\Models\Location::scopeOrderParent | ( | $query, | |
$order | |||
) |
Query builder scope to order on parent.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
Definition at line 163 of file Location.php.
App\Models\Location::scopeTextsearch | ( | $query, | |
$search | |||
) |
Query builder scope to search on text.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $search Search term
- Returns
- Illuminate Modified query builder
Definition at line 130 of file Location.php.
App\Models\Location::users | ( | ) |
Definition at line 43 of file Location.php.
Member Data Documentation
|
protected |
Definition at line 13 of file Location.php.
|
protected |
Definition at line 41 of file Location.php.
|
protected |
Definition at line 32 of file Location.php.
|
protected |
Initial value:
= array(
'name' => 'required|min:3|max:255|unique:locations,name,NULL,deleted_at',
'city' => 'min:3|max:255',
'state' => 'min:2|max:32',
'country' => 'min:2|max:2|max:2',
'address' => 'min:5|max:80',
'address2' => 'min:2|max:80',
'zip' => 'min:3|max:10',
)
Definition at line 15 of file Location.php.
|
protected |
Definition at line 14 of file Location.php.
The documentation for this class was generated from the following file: