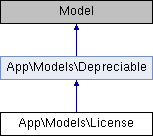
Public Member Functions | |
company () | |
assignedusers () | |
Get the assigned user. More... | |
assetlog () | |
Get asset logs for this asset. More... | |
uploads () | |
Get uploads for this asset. More... | |
adminuser () | |
Get admin user for this asset. More... | |
totalSeatsByLicenseID () | |
Get total licenses. More... | |
availcount () | |
Get the number of available seats. More... | |
assignedcount () | |
Get the number of assigned seats. More... | |
remaincount () | |
totalcount () | |
Get the total number of seats. More... | |
licenseseats () | |
Get license seat data. More... | |
supplier () | |
freeSeat () | |
scopeTextSearch ($query, $search) | |
Query builder scope to search on text. More... | |
depreciation () | |
Depreciation Relation, and associated helper methods. More... | |
get_depreciation () | |
getDepreciatedValue () | |
time_until_depreciated () | |
depreciated_date () | |
Static Public Member Functions | |
static | assetcount () |
Get total licenses. More... | |
static | availassetcount () |
Get total licenses not checked out. More... | |
static | getExpiringLicenses ($days=60) |
Public Attributes | |
$timestamps = true | |
Protected Attributes | |
$injectUniqueIdentifier = true | |
$dates = ['deleted_at'] | |
$guarded = 'id' | |
$table = 'licenses' | |
$rules | |
Detailed Description
Definition at line 10 of file License.php.
Member Function Documentation
App\Models\License::adminuser | ( | ) |
Get admin user for this asset.
Definition at line 73 of file License.php.
|
static |
Get total licenses.
Definition at line 81 of file License.php.
App\Models\License::assetlog | ( | ) |
Get asset logs for this asset.
Definition at line 50 of file License.php.
App\Models\License::assignedcount | ( | ) |
Get the number of assigned seats.
Definition at line 126 of file License.php.
App\Models\License::assignedusers | ( | ) |
Get the assigned user.
Definition at line 42 of file License.php.
|
static |
Get total licenses not checked out.
Definition at line 102 of file License.php.
App\Models\License::availcount | ( | ) |
Get the number of available seats.
Definition at line 113 of file License.php.
App\Models\License::company | ( | ) |
Definition at line 34 of file License.php.
|
inherited |
Definition at line 77 of file Depreciable.php.
|
inherited |
Depreciation Relation, and associated helper methods.
Definition at line 28 of file Depreciable.php.
App\Models\License::freeSeat | ( | ) |
Definition at line 172 of file License.php.
|
inherited |
Definition at line 33 of file Depreciable.php.
|
inherited |
- Returns
- float|int
Definition at line 42 of file Depreciable.php.
|
static |
Definition at line 182 of file License.php.
App\Models\License::licenseseats | ( | ) |
Get license seat data.
Definition at line 162 of file License.php.
App\Models\License::remaincount | ( | ) |
Definition at line 140 of file License.php.
App\Models\License::scopeTextSearch | ( | $query, | |
$search | |||
) |
Query builder scope to search on text.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $search Search term
- Returns
- Illuminate Modified query builder
Definition at line 202 of file License.php.
App\Models\License::supplier | ( | ) |
Definition at line 167 of file License.php.
|
inherited |
Definition at line 62 of file Depreciable.php.
App\Models\License::totalcount | ( | ) |
Get the total number of seats.
Definition at line 151 of file License.php.
App\Models\License::totalSeatsByLicenseID | ( | ) |
Get total licenses.
Definition at line 91 of file License.php.
App\Models\License::uploads | ( | ) |
Get uploads for this asset.
Definition at line 60 of file License.php.
Member Data Documentation
|
protected |
Definition at line 17 of file License.php.
|
protected |
Definition at line 21 of file License.php.
|
protected |
Definition at line 14 of file License.php.
|
protected |
Definition at line 23 of file License.php.
|
protected |
Definition at line 22 of file License.php.
App\Models\License::$timestamps = true |
Definition at line 19 of file License.php.
The documentation for this class was generated from the following file: