Model for Components. More...
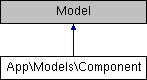
Public Member Functions | |
location () | |
assets () | |
admin () | |
company () | |
category () | |
assetlog () | |
Get action logs for this consumable. More... | |
numRemaining () | |
scopeTextSearch ($query, $search) | |
Query builder scope to search on text. More... | |
scopeOrderCategory ($query, $order) | |
Query builder scope to order on company. More... | |
scopeOrderLocation ($query, $order) | |
Query builder scope to order on company. More... | |
scopeOrderCompany ($query, $order) | |
Query builder scope to order on company. More... | |
Public Attributes | |
$rules | |
Category validation rules. More... | |
Protected Attributes | |
$dates = ['deleted_at'] | |
$table = 'components' | |
$injectUniqueIdentifier = true | |
$fillable = ['name','company_id','category_id'] | |
Detailed Description
Member Function Documentation
App\Models\Component::admin | ( | ) |
Definition at line 63 of file Component.php.
App\Models\Component::assetlog | ( | ) |
Get action logs for this consumable.
Definition at line 82 of file Component.php.
App\Models\Component::assets | ( | ) |
Definition at line 58 of file Component.php.
App\Models\Component::category | ( | ) |
Definition at line 74 of file Component.php.
App\Models\Component::company | ( | ) |
Definition at line 68 of file Component.php.
App\Models\Component::location | ( | ) |
Definition at line 53 of file Component.php.
App\Models\Component::numRemaining | ( | ) |
Definition at line 88 of file Component.php.
App\Models\Component::scopeOrderCategory | ( | $query, | |
$order | |||
) |
Query builder scope to order on company.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
Definition at line 152 of file Component.php.
App\Models\Component::scopeOrderCompany | ( | $query, | |
$order | |||
) |
Query builder scope to order on company.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
Definition at line 179 of file Component.php.
App\Models\Component::scopeOrderLocation | ( | $query, | |
$order | |||
) |
Query builder scope to order on company.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
Definition at line 165 of file Component.php.
App\Models\Component::scopeTextSearch | ( | $query, | |
$search | |||
) |
Query builder scope to search on text.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $search Search term
- Returns
- Illuminate Modified query builder Query builder scope to search on text
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $search Search term
- Returns
- Illuminate Modified query builder
Definition at line 119 of file Component.php.
Member Data Documentation
|
protected |
Definition at line 24 of file Component.php.
|
protected |
Definition at line 51 of file Component.php.
|
protected |
Definition at line 43 of file Component.php.
App\Models\Component::$rules |
Category validation rules.
Definition at line 31 of file Component.php.
|
protected |
Definition at line 25 of file Component.php.
The documentation for this class was generated from the following file: