Model for Assets. More...
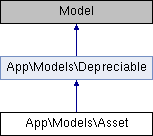
Public Member Functions | |
company () | |
checkOutToUser ($user, $admin, $checkout_at=null, $expected_checkin=null, $note=null, $name=null) | |
Checkout asset. More... | |
checkOutNotifyMail ($log_id, $user, $checkout_at, $expected_checkin, $note) | |
checkOutNotifySlack ($settings, $admin, $note=null) | |
validationRules ($id= '0') | |
createCheckoutLog ($checkout_at=null, $admin, $user, $expected_checkin=null, $note=null) | |
depreciation () | |
Set depreciation relationship. More... | |
get_depreciation () | |
Get depreciation attribute from associated asset model. More... | |
uploads () | |
Get uploads for this asset. More... | |
assigneduser () | |
assetloc () | |
Get the asset's location based on the assigned user. More... | |
defaultLoc () | |
Get the asset's location based on default RTD location. More... | |
assetlog () | |
Get action logs for this asset. More... | |
assetmaintenances () | |
assetmaintenances Get improvements for this asset More... | |
adminuser () | |
Get action logs for this asset. More... | |
assetstatus () | |
Get total assets. More... | |
showAssetName () | |
Get name for EULA. More... | |
warrantee_expires () | |
model () | |
licenses () | |
Get the license seat information. More... | |
licenseseats () | |
supplier () | |
months_until_eol () | |
eol_date () | |
checkin_email () | |
requireAcceptance () | |
getEula () | |
scopeHardware ($query) | |
BEGIN QUERY SCOPESMore... | |
scopePending ($query) | |
Query builder scope for pending assets. More... | |
scopeRTD ($query) | |
Query builder scope for RTD assets. More... | |
scopeUndeployable ($query) | |
Query builder scope for Undeployable assets. More... | |
scopeArchived ($query) | |
Query builder scope for Archived assets. More... | |
scopeDeployed ($query) | |
Query builder scope for Deployed assets. More... | |
scopeRequestableAssets ($query) | |
Query builder scope for Requestable assets. More... | |
scopeDeleted ($query) | |
Query builder scope for Deleted assets. More... | |
scopeInModelList ($query, array $modelIdListing) | |
scopeInModelList Get all assets in the provided listing of model ids More... | |
scopeNotYetAccepted ($query) | |
Query builder scope to get not-yet-accepted assets. More... | |
scopeRejected ($query) | |
Query builder scope to get rejected assets. More... | |
scopeAccepted ($query) | |
Query builder scope to get accepted assets. More... | |
scopeTextSearch ($query, $search) | |
Query builder scope to search on text for complex Bootstrap Tables API. More... | |
scopeOrderModels ($query, $order) | |
Query builder scope to order on model. More... | |
scopeOrderAssigned ($query, $order) | |
Query builder scope to order on assigned user. More... | |
scopeOrderStatus ($query, $order) | |
Query builder scope to order on status. More... | |
scopeOrderCompany ($query, $order) | |
Query builder scope to order on company. More... | |
scopeOrderCategory ($query, $order) | |
Query builder scope to order on category. More... | |
scopeOrderLocation ($query, $order) | |
Query builder scope to order on model. More... | |
getDepreciatedValue () | |
time_until_depreciated () | |
depreciated_date () | |
Static Public Member Functions | |
static | assetcount () |
Get total assets. More... | |
static | availassetcount () |
Get total assets not checked out. More... | |
static | getRequestable () |
Get requestable assets. More... | |
static | getExpiringWarrantee ($days=30) |
static | autoincrement_asset () |
Get auto-increment. More... | |
Protected Attributes | |
$table = 'assets' | |
$injectUniqueIdentifier = true | |
$rules | |
$fillable = ['name','model_id','status_id','asset_tag'] | |
Detailed Description
Member Function Documentation
App\Models\Asset::adminuser | ( | ) |
|
static |
App\Models\Asset::assetloc | ( | ) |
App\Models\Asset::assetlog | ( | ) |
App\Models\Asset::assetmaintenances | ( | ) |
App\Models\Asset::assetstatus | ( | ) |
App\Models\Asset::assigneduser | ( | ) |
|
static |
|
static |
App\Models\Asset::checkOutNotifyMail | ( | $log_id, | |
$user, | |||
$checkout_at, | |||
$expected_checkin, | |||
$note | |||
) |
Definition at line 114 of file Asset.php.
App\Models\Asset::checkOutNotifySlack | ( | $settings, | |
$admin, | |||
$note = null |
|||
) |
Definition at line 138 of file Asset.php.
App\Models\Asset::checkOutToUser | ( | $user, | |
$admin, | |||
$checkout_at = null , |
|||
$expected_checkin = null , |
|||
$note = null , |
|||
$name = null |
|||
) |
Checkout asset.
Definition at line 74 of file Asset.php.
App\Models\Asset::company | ( | ) |
App\Models\Asset::createCheckoutLog | ( | $checkout_at = null , |
|
$admin, | |||
$user, | |||
$expected_checkin = null , |
|||
$note = null |
|||
) |
App\Models\Asset::defaultLoc | ( | ) |
|
inherited |
Definition at line 77 of file Depreciable.php.
App\Models\Asset::depreciation | ( | ) |
App\Models\Asset::get_depreciation | ( | ) |
|
inherited |
- Returns
- float|int
Definition at line 42 of file Depreciable.php.
|
static |
|
static |
App\Models\Asset::licenses | ( | ) |
App\Models\Asset::licenseseats | ( | ) |
App\Models\Asset::model | ( | ) |
App\Models\Asset::scopeAccepted | ( | $query | ) |
App\Models\Asset::scopeArchived | ( | $query | ) |
Query builder scope for Archived assets.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeDeleted | ( | $query | ) |
App\Models\Asset::scopeDeployed | ( | $query | ) |
App\Models\Asset::scopeHardware | ( | $query | ) |
App\Models\Asset::scopeInModelList | ( | $query, | |
array | $modelIdListing | ||
) |
App\Models\Asset::scopeNotYetAccepted | ( | $query | ) |
App\Models\Asset::scopeOrderAssigned | ( | $query, | |
$order | |||
) |
Query builder scope to order on assigned user.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeOrderCategory | ( | $query, | |
$order | |||
) |
Query builder scope to order on category.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeOrderCompany | ( | $query, | |
$order | |||
) |
Query builder scope to order on company.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeOrderLocation | ( | $query, | |
$order | |||
) |
Query builder scope to order on model.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder TODO: Extend this method out for checked out assets as well. Right now it only checks the location name related to rtd_location_id
App\Models\Asset::scopeOrderModels | ( | $query, | |
$order | |||
) |
Query builder scope to order on model.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeOrderStatus | ( | $query, | |
$order | |||
) |
Query builder scope to order on status.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance text $order Order
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopePending | ( | $query | ) |
Query builder scope for pending assets.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeRejected | ( | $query | ) |
App\Models\Asset::scopeRequestableAssets | ( | $query | ) |
Query builder scope for Requestable assets.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeRTD | ( | $query | ) |
Query builder scope for RTD assets.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance
- Returns
- Illuminate Modified query builder
App\Models\Asset::scopeTextSearch | ( | $query, | |
$search | |||
) |
App\Models\Asset::scopeUndeployable | ( | $query | ) |
Query builder scope for Undeployable assets.
- Parameters
-
Illuminate\Database\Query\Builder $query Query builder instance
- Returns
- Illuminate Modified query builder
App\Models\Asset::supplier | ( | ) |
|
inherited |
Definition at line 62 of file Depreciable.php.
App\Models\Asset::uploads | ( | ) |
Get uploads for this asset.
App\Models\Asset::validationRules | ( | $id = '0' | ) |
App\Models\Asset::warrantee_expires | ( | ) |
Member Data Documentation
|
protected |
|
protected |
The documentation for this class was generated from the following file: