Model for the Actionlog (the table that keeps a historical log of checkouts, checkins, and updates). More...
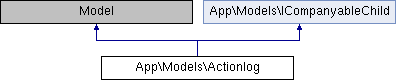
Public Member Functions | |
getCompanyableParents () | |
assetlog () | |
uploads () | |
licenselog () | |
accessorylog () | |
consumablelog () | |
adminlog () | |
userlog () | |
childlogs () | |
parentlog () | |
get_src ($type= 'assets') | |
Check if the file exists, and if it does, force a download. More... | |
logaction ($actiontype) | |
Get the parent category name. More... | |
getListingOfActionLogsChronologicalOrder () | |
getListingOfActionLogsChronologicalOrder More... | |
getLatestCheckoutActionForAssets () | |
getLatestCheckoutActionForAssets More... | |
scopeCheckoutWithoutAcceptance ($query) | |
scopeCheckoutWithoutAcceptance More... | |
Public Attributes | |
$timestamps = true | |
Protected Attributes | |
$dates = [ 'deleted_at' ] | |
$table = 'asset_logs' | |
$fillable = [ 'created_at', 'asset_type' ] | |
Detailed Description
Model for the Actionlog (the table that keeps a historical log of checkouts, checkins, and updates).
- Version
- v1.0
Definition at line 15 of file Actionlog.php.
Member Function Documentation
App\Models\Actionlog::accessorylog | ( | ) |
Definition at line 53 of file Actionlog.php.
App\Models\Actionlog::adminlog | ( | ) |
Definition at line 67 of file Actionlog.php.
App\Models\Actionlog::assetlog | ( | ) |
Definition at line 31 of file Actionlog.php.
App\Models\Actionlog::childlogs | ( | ) |
Definition at line 81 of file Actionlog.php.
App\Models\Actionlog::consumablelog | ( | ) |
Definition at line 60 of file Actionlog.php.
App\Models\Actionlog::get_src | ( | $type = 'assets' | ) |
Check if the file exists, and if it does, force a download.
Definition at line 96 of file Actionlog.php.
App\Models\Actionlog::getCompanyableParents | ( | ) |
Implements App\Models\ICompanyableChild.
Definition at line 26 of file Actionlog.php.
App\Models\Actionlog::getLatestCheckoutActionForAssets | ( | ) |
getLatestCheckoutActionForAssets
- Returns
- mixed
- Version
- v1.0
Definition at line 145 of file Actionlog.php.
App\Models\Actionlog::getListingOfActionLogsChronologicalOrder | ( | ) |
getListingOfActionLogsChronologicalOrder
- Returns
- mixed
- Version
- v1.0
Definition at line 127 of file Actionlog.php.
App\Models\Actionlog::licenselog | ( | ) |
Definition at line 46 of file Actionlog.php.
App\Models\Actionlog::logaction | ( | $actiontype | ) |
Get the parent category name.
Definition at line 108 of file Actionlog.php.
App\Models\Actionlog::parentlog | ( | ) |
Definition at line 87 of file Actionlog.php.
App\Models\Actionlog::scopeCheckoutWithoutAcceptance | ( | $query | ) |
scopeCheckoutWithoutAcceptance
- Parameters
-
$query
- Returns
- mixed
- Version
- v1.0
Definition at line 164 of file Actionlog.php.
App\Models\Actionlog::uploads | ( | ) |
Definition at line 38 of file Actionlog.php.
App\Models\Actionlog::userlog | ( | ) |
Definition at line 74 of file Actionlog.php.
Member Data Documentation
|
protected |
Definition at line 20 of file Actionlog.php.
|
protected |
Definition at line 24 of file Actionlog.php.
|
protected |
Definition at line 22 of file Actionlog.php.
App\Models\Actionlog::$timestamps = true |
Definition at line 23 of file Actionlog.php.
The documentation for this class was generated from the following file: