This controller handles all actions related to Users for the Snipe-IT Asset Management application. More...
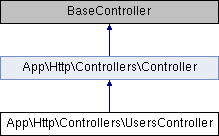
Public Member Functions | |
getIndex () | |
Returns a view that invokes the ajax tables which actually contains the content for the users listing, which is generated in getDatatable(). More... | |
getCreate () | |
Returns a view that displays the user creation form. More... | |
postCreate (SetupUserRequest $request) | |
Validate and store the new user data, or return an error. More... | |
store () | |
JSON handler for creating a user through a modal. More... | |
getEdit ($id=null) | |
Returns a view that displays the edit user form. More... | |
postEdit ($id=null) | |
Validate and save edited user data from edit form. More... | |
getDelete ($id=null) | |
Delete a user. More... | |
postBulkEdit () | |
Returns a view that confirms the user's a bulk delete will be applied to. More... | |
postBulkSave () | |
Soft-delete bulk users. More... | |
getRestore ($id=null) | |
Restore a deleted user. More... | |
getView ($userId=null) | |
Return a view with user detail. More... | |
getUnsuspend ($id=null) | |
Unsuspend a user. More... | |
getClone ($id=null) | |
Return a view containing a pre-populated new user form, populated with some fields from an existing user. More... | |
getImport () | |
Return user import view. More... | |
postImport () | |
Handle user import file. More... | |
getDatatable ($status=null) | |
Return JSON response with a list of user details for the getIndex() view. More... | |
postUpload (AssetFileRequest $request, $userId=null) | |
Return JSON response with a list of user details for the getIndex() view. More... | |
getDeleteFile ($userId=null, $fileId=null) | |
Delete file. More... | |
displayFile ($userId=null, $fileId=null) | |
Display/download the uploaded file. More... | |
getLDAP () | |
Return view for LDAP import. More... | |
postLDAP () | |
LDAP form processing. More... | |
getAssetList ($userId) | |
Return JSON containing a list of assets assigned to a user. More... | |
Protected Attributes | |
$ldapValidationRules | |
Detailed Description
This controller handles all actions related to Users for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 38 of file UsersController.php.
Member Function Documentation
App\Http\Controllers\UsersController::displayFile | ( | $userId = null , |
|
$fileId = null |
|||
) |
Display/download the uploaded file.
- Since
- [v1.6]
- Parameters
-
int $userId int $fileId
- Returns
- mixed
Definition at line 1017 of file UsersController.php.
App\Http\Controllers\UsersController::getAssetList | ( | $userId | ) |
Return JSON containing a list of assets assigned to a user.
- Since
- [v3.0]
- Returns
- string JSON
Definition at line 1251 of file UsersController.php.
App\Http\Controllers\UsersController::getClone | ( | $id = null | ) |
Return a view containing a pre-populated new user form, populated with some fields from an existing user.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- Redirect
Definition at line 608 of file UsersController.php.
App\Http\Controllers\UsersController::getCreate | ( | ) |
Returns a view that displays the user creation form.
- Since
- [v1.0]
- Returns
- View
Definition at line 63 of file UsersController.php.
App\Http\Controllers\UsersController::getDatatable | ( | $status = null | ) |
Return JSON response with a list of user details for the getIndex() view.
- Since
- [v1.6]
- See also
- UsersController::getIndex() method that consumed this JSON response
- Returns
- string JSON
Definition at line 805 of file UsersController.php.
App\Http\Controllers\UsersController::getDelete | ( | $id = null | ) |
Delete a user.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- Redirect
Definition at line 329 of file UsersController.php.
App\Http\Controllers\UsersController::getDeleteFile | ( | $userId = null , |
|
$fileId = null |
|||
) |
Delete file.
- Since
- [v1.6]
- Parameters
-
int $userId int $fileId
- Returns
- Redirect
Definition at line 980 of file UsersController.php.
App\Http\Controllers\UsersController::getEdit | ( | $id = null | ) |
Returns a view that displays the edit user form.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- View
Definition at line 183 of file UsersController.php.
App\Http\Controllers\UsersController::getImport | ( | ) |
Return user import view.
- Since
- [v1.0]
- Returns
- View
Definition at line 674 of file UsersController.php.
App\Http\Controllers\UsersController::getIndex | ( | ) |
Returns a view that invokes the ajax tables which actually contains the content for the users listing, which is generated in getDatatable().
- See also
- UsersController::getDatatable() method that generates the JSON response
- Since
- [v1.0]
- Returns
- View
Definition at line 51 of file UsersController.php.
App\Http\Controllers\UsersController::getLDAP | ( | ) |
Return view for LDAP import.
- Since
- [v1.8]
- Returns
- View
Definition at line 1047 of file UsersController.php.
App\Http\Controllers\UsersController::getRestore | ( | $id = null | ) |
Restore a deleted user.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- Redirect
Definition at line 496 of file UsersController.php.
App\Http\Controllers\UsersController::getUnsuspend | ( | $id = null | ) |
Unsuspend a user.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- Redirect
Definition at line 563 of file UsersController.php.
App\Http\Controllers\UsersController::getView | ( | $userId = null | ) |
Return a view with user detail.
- Since
- [v1.0]
- Parameters
-
int $userId
- Returns
- View
Definition at line 532 of file UsersController.php.
App\Http\Controllers\UsersController::postBulkEdit | ( | ) |
Returns a view that confirms the user's a bulk delete will be applied to.
- Since
- [v1.7]
- Returns
- View
Definition at line 387 of file UsersController.php.
App\Http\Controllers\UsersController::postBulkSave | ( | ) |
Soft-delete bulk users.
- Since
- [v1.0]
- Returns
- Redirect
PhpUnreachableStatementInspection Known to be unreachable but kept following discussion: https://github.com/snipe/snipe-it/pull/1423
Definition at line 410 of file UsersController.php.
App\Http\Controllers\UsersController::postCreate | ( | SetupUserRequest | $request | ) |
Validate and store the new user data, or return an error.
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 99 of file UsersController.php.
App\Http\Controllers\UsersController::postEdit | ( | $id = null | ) |
Validate and save edited user data from edit form.
- Since
- [v1.0]
- Parameters
-
int $id
- Returns
- Redirect
Definition at line 240 of file UsersController.php.
App\Http\Controllers\UsersController::postImport | ( | ) |
Handle user import file.
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 697 of file UsersController.php.
App\Http\Controllers\UsersController::postLDAP | ( | ) |
LDAP form processing.
- Since
- [v1.8]
- Returns
- Redirect
Definition at line 1095 of file UsersController.php.
App\Http\Controllers\UsersController::postUpload | ( | AssetFileRequest | $request, |
$userId = null |
|||
) |
Return JSON response with a list of user details for the getIndex() view.
- Since
- [v1.6]
- Parameters
-
int $userId
- Returns
- string JSON
Definition at line 930 of file UsersController.php.
App\Http\Controllers\UsersController::store | ( | ) |
JSON handler for creating a user through a modal.
- Since
- [v1.8]
- Returns
- string JSON
Definition at line 141 of file UsersController.php.
Member Data Documentation
|
protected |
Definition at line 1080 of file UsersController.php.
The documentation for this class was generated from the following file: