This controller handles all actions related to Settings for the Snipe-IT Asset Management application. More...
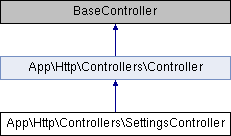
Public Member Functions | |
getSetupIndex () | |
Checks to see whether or not the database has a migrations table and a user, otherwise display the setup view. More... | |
ajaxTestEmail () | |
Test the email configuration. More... | |
postSaveFirstAdmin (SetupUserRequest $request) | |
Save the first admin user from Setup. More... | |
getSetupUser () | |
Return the admin user creation form in Setup. More... | |
getSetupDone () | |
Return the view that tells the user that the Setup is done. More... | |
getSetupMigrate () | |
Migrate the database tables, and return the output to a view for Setup. More... | |
getIndex () | |
Return a view that shows some of the key settings. More... | |
getEdit () | |
Return a form to allow a super admin to update settings. More... | |
postEdit (SettingRequest $request) | |
Validate and process settings edit form. More... | |
getBackups () | |
Show the listing of backups. More... | |
postBackups () | |
Process the backup. More... | |
downloadFile ($filename=null) | |
Download the backup file. More... | |
deleteFile ($filename=null) | |
Delete the backup file. More... | |
Detailed Description
This controller handles all actions related to Settings for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 27 of file SettingsController.php.
Member Function Documentation
App\Http\Controllers\SettingsController::ajaxTestEmail | ( | ) |
Test the email configuration.
- Since
- [v3.0]
- Returns
- Redirect
Definition at line 136 of file SettingsController.php.
App\Http\Controllers\SettingsController::deleteFile | ( | $filename = null | ) |
Delete the backup file.
- Since
- [v1.8]
- Returns
- View
Definition at line 502 of file SettingsController.php.
App\Http\Controllers\SettingsController::downloadFile | ( | $filename = null | ) |
Download the backup file.
- Since
- [v1.8]
- Returns
- Redirect
Definition at line 475 of file SettingsController.php.
App\Http\Controllers\SettingsController::getBackups | ( | ) |
Show the listing of backups.
- Since
- [v1.8]
- Returns
- View
Definition at line 416 of file SettingsController.php.
App\Http\Controllers\SettingsController::getEdit | ( | ) |
Return a form to allow a super admin to update settings.
- Since
- [v1.0]
- Returns
- View
Definition at line 272 of file SettingsController.php.
App\Http\Controllers\SettingsController::getIndex | ( | ) |
Return a view that shows some of the key settings.
- Since
- [v1.0]
- Returns
- View
Definition at line 255 of file SettingsController.php.
App\Http\Controllers\SettingsController::getSetupDone | ( | ) |
Return the view that tells the user that the Setup is done.
- Since
- [v3.0]
- Returns
- View
Definition at line 219 of file SettingsController.php.
App\Http\Controllers\SettingsController::getSetupIndex | ( | ) |
Checks to see whether or not the database has a migrations table and a user, otherwise display the setup view.
- Since
- [v3.0]
- Returns
- View
Definition at line 38 of file SettingsController.php.
App\Http\Controllers\SettingsController::getSetupMigrate | ( | ) |
Migrate the database tables, and return the output to a view for Setup.
- Since
- [v3.0]
- Returns
- View
Definition at line 234 of file SettingsController.php.
App\Http\Controllers\SettingsController::getSetupUser | ( | ) |
Return the admin user creation form in Setup.
- Since
- [v3.0]
- Returns
- View
Definition at line 205 of file SettingsController.php.
App\Http\Controllers\SettingsController::postBackups | ( | ) |
Process the backup.
- Since
- [v1.8]
- Returns
- Redirect
Definition at line 454 of file SettingsController.php.
App\Http\Controllers\SettingsController::postEdit | ( | SettingRequest | $request | ) |
Validate and process settings edit form.
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 288 of file SettingsController.php.
App\Http\Controllers\SettingsController::postSaveFirstAdmin | ( | SetupUserRequest | $request | ) |
Save the first admin user from Setup.
- Since
- [v3.0]
- Returns
- Redirect
Definition at line 158 of file SettingsController.php.
The documentation for this class was generated from the following file: