This controller handles all actions related to Licenses for the Snipe-IT Asset Management application. More...
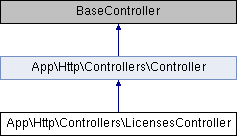
Public Member Functions | |
getIndex () | |
Returns a view that invokes the ajax tables which actually contains the content for the licenses listing, which is generated in getDatatable. More... | |
getCreate () | |
Returns a form view that allows an admin to create a new licence. More... | |
postCreate () | |
Validates and stores the license form data submitted from the new license form. More... | |
getEdit ($licenseId=null) | |
Returns a form with existing license data to allow an admin to update license information. More... | |
postEdit ($licenseId=null) | |
Validates and stores the license form data submitted from the edit license form. More... | |
getDelete ($licenseId) | |
Checks to see whether the selected license can be deleted, and if it can, marks it as deleted. More... | |
getCheckout ($seatId) | |
Provides the form view for checking out a license to a user. More... | |
postCheckout ($seatId) | |
Validates and stores the license checkout action. More... | |
getCheckin ($seatId=null, $backto=null) | |
Makes the form view to check a license seat back into inventory. More... | |
postCheckin ($seatId=null, $backto=null) | |
Validates and stores the license checkin action. More... | |
getView ($licenseId=null) | |
Makes the license detail page. More... | |
getClone ($licenseId=null) | |
postUpload ($licenseId=null) | |
Validates and stores files associated with a license. More... | |
getDeleteFile ($licenseId=null, $fileId=null) | |
Deletes the selected license file. More... | |
displayFile ($licenseId=null, $fileId=null) | |
Allows the selected file to be viewed. More... | |
getDatatable () | |
Generates a JSON response to populate the licence index datatables. More... | |
getFreeLicense ($licenseId) | |
Generates the next free seat ID for checkout. More... | |
Detailed Description
This controller handles all actions related to Licenses for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 33 of file LicensesController.php.
Member Function Documentation
App\Http\Controllers\LicensesController::displayFile | ( | $licenseId = null , |
|
$fileId = null |
|||
) |
Allows the selected file to be viewed.
- Since
- [v1.4]
- Parameters
-
int $licenseId int $fileId
- Returns
- Redirect
Definition at line 959 of file LicensesController.php.
App\Http\Controllers\LicensesController::getCheckin | ( | $seatId = null , |
|
$backto = null |
|||
) |
Makes the form view to check a license seat back into inventory.
- Since
- [v1.0]
- Parameters
-
int $seatId string $backto
- Returns
- View
Definition at line 641 of file LicensesController.php.
App\Http\Controllers\LicensesController::getCheckout | ( | $seatId | ) |
Provides the form view for checking out a license to a user.
Here we pass the license seat ID instead of the license ID, because licenses themselves are never checked out to anyone, only the seats associated with them.
- Since
- [v1.0]
- Parameters
-
int $seatId
- Returns
- View
Definition at line 427 of file LicensesController.php.
App\Http\Controllers\LicensesController::getClone | ( | $licenseId = null | ) |
Definition at line 799 of file LicensesController.php.
App\Http\Controllers\LicensesController::getCreate | ( | ) |
Returns a form view that allows an admin to create a new licence.
- See also
- AccessoriesController::getDatatable() method that generates the JSON response
- Since
- [v1.0]
- Returns
- View
Definition at line 60 of file LicensesController.php.
App\Http\Controllers\LicensesController::getDatatable | ( | ) |
Generates a JSON response to populate the licence index datatables.
- See also
- LicensesController::getIndex() method that provides the view
- Since
- [v1.0]
- Returns
- String JSON
Definition at line 992 of file LicensesController.php.
App\Http\Controllers\LicensesController::getDelete | ( | $licenseId | ) |
Checks to see whether the selected license can be deleted, and if it can, marks it as deleted.
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- Redirect
Definition at line 379 of file LicensesController.php.
App\Http\Controllers\LicensesController::getDeleteFile | ( | $licenseId = null , |
|
$fileId = null |
|||
) |
Deletes the selected license file.
- Since
- [v1.0]
- Parameters
-
int $licenseId int $fileId
- Returns
- Redirect
Definition at line 918 of file LicensesController.php.
App\Http\Controllers\LicensesController::getEdit | ( | $licenseId = null | ) |
Returns a form with existing license data to allow an admin to update license information.
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- View
Definition at line 186 of file LicensesController.php.
App\Http\Controllers\LicensesController::getFreeLicense | ( | $licenseId | ) |
Generates the next free seat ID for checkout.
- Todo:
- This is a dumb way to solve this problem. Author should refactor. And go hide in a hole and think about what she's done. And perhaps find a new line of work. And get in the sea.
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- View
Definition at line 1051 of file LicensesController.php.
App\Http\Controllers\LicensesController::getIndex | ( | ) |
Returns a view that invokes the ajax tables which actually contains the content for the licenses listing, which is generated in getDatatable.
- See also
- LicensesController::getDatatable() method that generates the JSON response
- Since
- [v1.0]
- Returns
- View
Definition at line 45 of file LicensesController.php.
App\Http\Controllers\LicensesController::getView | ( | $licenseId = null | ) |
Makes the license detail page.
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- View
Definition at line 778 of file LicensesController.php.
App\Http\Controllers\LicensesController::postCheckin | ( | $seatId = null , |
|
$backto = null |
|||
) |
Validates and stores the license checkin action.
- See also
- LicensesController::getCheckin() method that provides the form view
- Since
- [v1.0]
- Parameters
-
int $seatId string $backto
- Returns
- Redirect
Definition at line 666 of file LicensesController.php.
App\Http\Controllers\LicensesController::postCheckout | ( | $seatId | ) |
Validates and stores the license checkout action.
- Todo:
- Switch to using a FormRequest for validation here.
- Since
- [v1.0]
- Parameters
-
int $seatId
- Returns
- Redirect
Definition at line 486 of file LicensesController.php.
App\Http\Controllers\LicensesController::postCreate | ( | ) |
Validates and stores the license form data submitted from the new license form.
- See also
- LicensesController::getCreate() method that provides the form view
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 87 of file LicensesController.php.
App\Http\Controllers\LicensesController::postEdit | ( | $licenseId = null | ) |
Validates and stores the license form data submitted from the edit license form.
- See also
- LicensesController::getEdit() method that provides the form view
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- Redirect
Definition at line 230 of file LicensesController.php.
App\Http\Controllers\LicensesController::postUpload | ( | $licenseId = null | ) |
Validates and stores files associated with a license.
- Todo:
- Switch to using the AssetFileRequest form request validator.
- Since
- [v1.0]
- Parameters
-
int $licenseId
- Returns
- Redirect
Definition at line 841 of file LicensesController.php.
The documentation for this class was generated from the following file: