This controller handles all actions related to User Groups for the Snipe-IT Asset Management application. More...
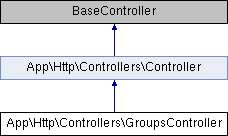
Public Member Functions | |
getIndex () | |
Returns a view that invokes the ajax tables which actually contains the content for the user group listing, which is generated in getDatatable. More... | |
getCreate () | |
Returns a view that displays a form to create a new User Group. More... | |
postCreate () | |
Validates and stores the new User Group data. More... | |
getEdit ($id=null) | |
Returns a view that presents a form to edit a User Group. More... | |
postEdit ($id=null) | |
Validates and stores the updated User Group data. More... | |
getDelete ($id=null) | |
Validates and deletes the User Group. More... | |
getDatatable () | |
Generates the JSON used to display the User Group listing. More... | |
Detailed Description
This controller handles all actions related to User Groups for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 19 of file GroupsController.php.
Member Function Documentation
App\Http\Controllers\GroupsController::getCreate | ( | ) |
Returns a view that displays a form to create a new User Group.
- See also
- GroupsController::postCreate()
- Since
- [v1.0]
- Returns
- View
Definition at line 44 of file GroupsController.php.
App\Http\Controllers\GroupsController::getDatatable | ( | ) |
Generates the JSON used to display the User Group listing.
- Since
- [v2.0]
- Returns
- String JSON
Definition at line 174 of file GroupsController.php.
App\Http\Controllers\GroupsController::getDelete | ( | $id = null | ) |
Validates and deletes the User Group.
- See also
- GroupsController::getEdit()
- Parameters
-
int $id
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 145 of file GroupsController.php.
App\Http\Controllers\GroupsController::getEdit | ( | $id = null | ) |
Returns a view that presents a form to edit a User Group.
- See also
- GroupsController::postEdit()
- Parameters
-
int $id
- Since
- [v1.0]
- Returns
- View
Definition at line 92 of file GroupsController.php.
App\Http\Controllers\GroupsController::getIndex | ( | ) |
Returns a view that invokes the ajax tables which actually contains the content for the user group listing, which is generated in getDatatable.
- See also
- GroupsController::getDatatable() method that generates the JSON response
- Since
- [v1.0]
- Returns
- View
Definition at line 30 of file GroupsController.php.
App\Http\Controllers\GroupsController::postCreate | ( | ) |
Validates and stores the new User Group data.
- See also
- GroupsController::getCreate()
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 65 of file GroupsController.php.
App\Http\Controllers\GroupsController::postEdit | ( | $id = null | ) |
Validates and stores the updated User Group data.
- See also
- GroupsController::getEdit()
- Parameters
-
int $id
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 112 of file GroupsController.php.
The documentation for this class was generated from the following file: