This class controls all actions related to Components for the Snipe-IT Asset Management application. More...
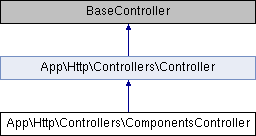
Public Member Functions | |
getIndex () | |
Returns a view that invokes the ajax tables which actually contains the content for the components listing, which is generated in getDatatable. More... | |
getCreate () | |
Returns a form to create a new component. More... | |
postCreate () | |
Validate and store data for new component. More... | |
getEdit ($componentId=null) | |
Return a view to edit a component. More... | |
postEdit ($componentId=null) | |
Return a view to edit a component. More... | |
getDelete ($componentId) | |
Delete a component. More... | |
postBulk ($componentId=null) | |
postBulkSave ($componentId=null) | |
getView ($componentId=null) | |
Return a view to display component information. More... | |
getCheckout ($componentId) | |
Returns a view that allows the checkout of a component to an asset. More... | |
postCheckout (ComponentCheckoutRequest $request, $componentId) | |
Validate and store checkout data. More... | |
getDatatable () | |
Generates the JSON response for accessories listing view. More... | |
getDataView ($componentId) | |
Return JSON data to populate the components view,. More... | |
Detailed Description
This class controls all actions related to Components for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 29 of file ComponentsController.php.
Member Function Documentation
App\Http\Controllers\ComponentsController::getCheckout | ( | $componentId | ) |
Returns a view that allows the checkout of a component to an asset.
- See also
- ComponentsController::postCheckout() method that stores the data.
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- View
Definition at line 279 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getCreate | ( | ) |
Returns a form to create a new component.
- See also
- ComponentsController::postCreate() method that stores the data
- Since
- [v3.0]
- Returns
- View
Definition at line 54 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getDatatable | ( | ) |
Generates the JSON response for accessories listing view.
For debugging, see at /api/accessories/list
- Since
- [v3.0]
- Returns
- string JSON
Definition at line 394 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getDataView | ( | $componentId | ) |
Return JSON data to populate the components view,.
- See also
- ComponentsController::getView() method that returns the view.
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- string JSON
Definition at line 475 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getDelete | ( | $componentId | ) |
Delete a component.
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- Redirect
Definition at line 210 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getEdit | ( | $componentId = null | ) |
Return a view to edit a component.
- See also
- ComponentsController::postEdit() method that stores the data.
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- View
Definition at line 126 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getIndex | ( | ) |
Returns a view that invokes the ajax tables which actually contains the content for the components listing, which is generated in getDatatable.
- See also
- ComponentsController::getDatatable() method that generates the JSON response
- Since
- [v3.0]
- Returns
- View
Definition at line 40 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::getView | ( | $componentId = null | ) |
Return a view to display component information.
- See also
- ComponentsController::getDataView() method that generates the JSON response
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- View
Definition at line 247 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::postBulk | ( | $componentId = null | ) |
Definition at line 227 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::postBulkSave | ( | $componentId = null | ) |
Definition at line 232 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::postCheckout | ( | ComponentCheckoutRequest | $request, |
$componentId | |||
) |
Validate and store checkout data.
- See also
- ComponentsController::getCheckout() method that returns the form.
- Since
- [v3.0]
- Parameters
-
int $componentId
- Returns
- Redirect
Definition at line 305 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::postCreate | ( | ) |
Validate and store data for new component.
- See also
- ComponentsController::getCreate() method that generates the view
- Since
- [v3.0]
- Returns
- Redirect
Definition at line 77 of file ComponentsController.php.
App\Http\Controllers\ComponentsController::postEdit | ( | $componentId = null | ) |
Return a view to edit a component.
- See also
- ComponentsController::getEdit() method presents the form.
- Parameters
-
int $componentId
- Since
- [v3.0]
- Returns
- Redirect
Definition at line 156 of file ComponentsController.php.
The documentation for this class was generated from the following file: