This controller handles all actions related to Companies for the Snipe-IT Asset Management application. More...
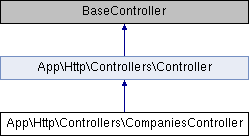
Public Member Functions | |
getIndex () | |
Returns view to display listing of companies. More... | |
getCreate () | |
Returns view to create a new company. More... | |
postCreate () | |
Save data from new company form. More... | |
getEdit ($companyId) | |
Return form to edit existing company. More... | |
postEdit ($companyId) | |
Save data from edit company form. More... | |
postDelete ($companyId) | |
Delete company. More... | |
Detailed Description
This controller handles all actions related to Companies for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 17 of file CompaniesController.php.
Member Function Documentation
App\Http\Controllers\CompaniesController::getCreate | ( | ) |
Returns view to create a new company.
- Since
- [v1.8]
- Returns
- View
Definition at line 39 of file CompaniesController.php.
App\Http\Controllers\CompaniesController::getEdit | ( | $companyId | ) |
Return form to edit existing company.
- Since
- [v1.8]
- Parameters
-
int $companyId
- Returns
- View
Definition at line 75 of file CompaniesController.php.
App\Http\Controllers\CompaniesController::getIndex | ( | ) |
Returns view to display listing of companies.
- Since
- [v1.8]
- Returns
- View
Definition at line 27 of file CompaniesController.php.
App\Http\Controllers\CompaniesController::postCreate | ( | ) |
Save data from new company form.
- Since
- [v1.8]
- Returns
- Redirect
Definition at line 51 of file CompaniesController.php.
App\Http\Controllers\CompaniesController::postDelete | ( | $companyId | ) |
Delete company.
- Since
- [v1.8]
- Parameters
-
int $companyId
- Returns
- Redirect
Definition at line 121 of file CompaniesController.php.
App\Http\Controllers\CompaniesController::postEdit | ( | $companyId | ) |
Save data from edit company form.
- Since
- [v1.8]
- Parameters
-
int $companyId
- Returns
- Redirect
Definition at line 93 of file CompaniesController.php.
The documentation for this class was generated from the following file: