This class controls all actions related to assets for the Snipe-IT Asset Management application. More...
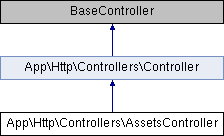
Public Member Functions | |
__construct () | |
getIndex () | |
Returns a view that invokes the ajax tables which actually contains the content for the assets listing, which is generated in getDatatable. More... | |
getCreate ($model_id=null) | |
Returns a view that presents a form to create a new asset. More... | |
postCreate (AssetRequest $request) | |
Validate and process new asset form data. More... | |
getEdit ($assetId=null) | |
Returns a view that presents a form to edit an existing asset. More... | |
postEdit ($assetId=null) | |
Validate and process asset edit form. More... | |
getDelete ($assetId) | |
Delete a given asset (mark as deleted). More... | |
getCheckout ($assetId) | |
Returns a view that presents a form to check an asset out to a user. More... | |
postCheckout (AssetCheckoutRequest $request, $assetId) | |
Validate and process the form data to check out an asset to a user. More... | |
getCheckin ($assetId, $backto=null) | |
Returns a view that presents a form to check an asset back into inventory. More... | |
postCheckin (AssetCheckinRequest $request, $assetId=null, $backto=null) | |
Validate and process the form data to check an asset back into inventory. More... | |
getView ($assetId=null) | |
Returns a view that presents information about an asset for detail view. More... | |
getQrCode ($assetId=null) | |
Return a QR code for the asset. More... | |
getImportUpload () | |
Get the Asset import upload page. More... | |
postAPIImportUpload (AssetFileRequest $request) | |
Upload the import file via AJAX. More... | |
getProcessImportFile ($filename) | |
Process the uploaded file. More... | |
getClone ($assetId=null) | |
Returns a view that presents a form to clone an asset. More... | |
getRestore ($assetId=null) | |
Retore a deleted asset. More... | |
postUpload (AssetFileRequest $request, $assetId=null) | |
Upload a file to the server. More... | |
getDeleteFile ($assetId=null, $fileId=null) | |
Delete the associated file. More... | |
displayFile ($assetId=null, $fileId=null) | |
Check for permissions and display the file. More... | |
postBulkEdit ($assets=null) | |
Display the bulk edit page. More... | |
postBulkSave ($assets=null) | |
Save bulk edits. More... | |
postBulkDelete ($assets=null) | |
Save bulk deleted. More... | |
getDatatable ($status=null) | |
Generates the JSON used to display the asset listing. More... | |
Protected Attributes | |
$qrCodeDimensions = array( 'height' => 3.5, 'width' => 3.5) | |
$barCodeDimensions = array( 'height' => 2, 'width' => 22) | |
Detailed Description
This class controls all actions related to assets for the Snipe-IT Asset Management application.
- Version
- v1.0
Definition at line 50 of file AssetsController.php.
Constructor & Destructor Documentation
App\Http\Controllers\AssetsController::__construct | ( | ) |
Definition at line 56 of file AssetsController.php.
Member Function Documentation
App\Http\Controllers\AssetsController::displayFile | ( | $assetId = null , |
|
$fileId = null |
|||
) |
Check for permissions and display the file.
- Parameters
-
int $assetId int $fileId
- Since
- [v1.0]
- Returns
- View
Definition at line 1036 of file AssetsController.php.
App\Http\Controllers\AssetsController::getCheckin | ( | $assetId, | |
$backto = null |
|||
) |
Returns a view that presents a form to check an asset back into inventory.
- Parameters
-
int $assetId string $backto
- Since
- [v1.0]
- Returns
- View
Definition at line 505 of file AssetsController.php.
App\Http\Controllers\AssetsController::getCheckout | ( | $assetId | ) |
Returns a view that presents a form to check an asset out to a user.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- View
Definition at line 433 of file AssetsController.php.
App\Http\Controllers\AssetsController::getClone | ( | $assetId = null | ) |
Returns a view that presents a form to clone an asset.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- View
Definition at line 854 of file AssetsController.php.
App\Http\Controllers\AssetsController::getCreate | ( | $model_id = null | ) |
Returns a view that presents a form to create a new asset.
- Since
- [v1.0]
- Returns
- View
Definition at line 84 of file AssetsController.php.
App\Http\Controllers\AssetsController::getDatatable | ( | $status = null | ) |
Generates the JSON used to display the asset listing.
- Parameters
-
string $status
- Since
- [v2.0]
- Returns
- String JSON
Definition at line 1322 of file AssetsController.php.
App\Http\Controllers\AssetsController::getDelete | ( | $assetId | ) |
Delete a given asset (mark as deleted).
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 399 of file AssetsController.php.
App\Http\Controllers\AssetsController::getDeleteFile | ( | $assetId = null , |
|
$fileId = null |
|||
) |
Delete the associated file.
- Parameters
-
int $assetId int $fileId
- Since
- [v1.0]
- Returns
- View
Definition at line 995 of file AssetsController.php.
App\Http\Controllers\AssetsController::getEdit | ( | $assetId = null | ) |
Returns a view that presents a form to edit an existing asset.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- View
Definition at line 232 of file AssetsController.php.
App\Http\Controllers\AssetsController::getImportUpload | ( | ) |
Get the Asset import upload page.
- Since
- [v2.0]
- Returns
- View
Definition at line 729 of file AssetsController.php.
App\Http\Controllers\AssetsController::getIndex | ( | ) |
Returns a view that invokes the ajax tables which actually contains the content for the assets listing, which is generated in getDatatable.
- See also
- AssetController::getDatatable() method that generates the JSON response
- Since
- [v1.0]
- Returns
- View
Definition at line 72 of file AssetsController.php.
App\Http\Controllers\AssetsController::getProcessImportFile | ( | $filename | ) |
Process the uploaded file.
- Parameters
-
string $filename
- Since
- [v2.0]
- Returns
- Redirect
Definition at line 827 of file AssetsController.php.
App\Http\Controllers\AssetsController::getQrCode | ( | $assetId = null | ) |
Return a QR code for the asset.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Response
Definition at line 699 of file AssetsController.php.
App\Http\Controllers\AssetsController::getRestore | ( | $assetId = null | ) |
Retore a deleted asset.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- View
Definition at line 904 of file AssetsController.php.
App\Http\Controllers\AssetsController::getView | ( | $assetId = null | ) |
Returns a view that presents information about an asset for detail view.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- View
Definition at line 649 of file AssetsController.php.
App\Http\Controllers\AssetsController::postAPIImportUpload | ( | AssetFileRequest | $request | ) |
Upload the import file via AJAX.
- Since
- [v2.0]
- Returns
- View
Definition at line 769 of file AssetsController.php.
App\Http\Controllers\AssetsController::postBulkDelete | ( | $assets = null | ) |
Save bulk deleted.
- Parameters
-
array $assets
- Since
- [v2.0]
- Returns
- View
Definition at line 1269 of file AssetsController.php.
App\Http\Controllers\AssetsController::postBulkEdit | ( | $assets = null | ) |
Display the bulk edit page.
- Parameters
-
int $assetId
- Since
- [v2.0]
- Returns
- View
Definition at line 1081 of file AssetsController.php.
App\Http\Controllers\AssetsController::postBulkSave | ( | $assets = null | ) |
Save bulk edits.
- Parameters
-
array $assets
- Since
- [v2.0]
- Returns
- Redirect
Definition at line 1165 of file AssetsController.php.
App\Http\Controllers\AssetsController::postCheckin | ( | AssetCheckinRequest | $request, |
$assetId = null , |
|||
$backto = null |
|||
) |
Validate and process the form data to check an asset back into inventory.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 527 of file AssetsController.php.
App\Http\Controllers\AssetsController::postCheckout | ( | AssetCheckoutRequest | $request, |
$assetId | |||
) |
Validate and process the form data to check out an asset to a user.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 458 of file AssetsController.php.
App\Http\Controllers\AssetsController::postCreate | ( | AssetRequest | $request | ) |
Validate and process new asset form data.
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 124 of file AssetsController.php.
App\Http\Controllers\AssetsController::postEdit | ( | $assetId = null | ) |
Validate and process asset edit form.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 274 of file AssetsController.php.
App\Http\Controllers\AssetsController::postUpload | ( | AssetFileRequest | $request, |
$assetId = null |
|||
) |
Upload a file to the server.
- Parameters
-
int $assetId
- Since
- [v1.0]
- Returns
- Redirect
Definition at line 938 of file AssetsController.php.
Member Data Documentation
|
protected |
Definition at line 53 of file AssetsController.php.
|
protected |
Definition at line 52 of file AssetsController.php.
The documentation for this class was generated from the following file: